I've been making boards in KiCad for a while now. I really enjoy figuring out how to route all the components in the PCB editor, especially the weird "hard" things like differential high speed signals. I'm probably not very good at it but so far the margins for the stuff I'm designing is wide enough that it works anyway.
Every time I'm working on this I feel like this would make a neat puzzle game, but I don't feel like building an entire PCB editor in a game engine to make this concept work. So I took a page from the Minecraft book and turned it into cubes. Making the problem discrete into a grid makes it way easier to reason about and a more logical game.
To make the whole thing easy to build I decided to go with a simple browser game. No need to deal with much platform support there and I don't have to either use one of the big game engines or write one myself. I debated for a few seconds whether I should go with TypeScript or just vanilla JS. In the end I wrote the entire thing in plain javascript.
The game
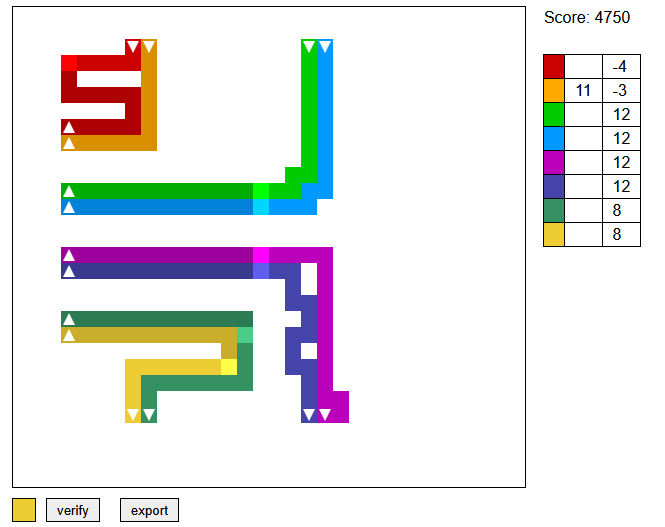
The whole game area is drawn as a element and the javascript code generates the DOM elements for a few of the user interface elements.
The goal of the game is to connect all the source blocks to the sink blocks of the same color. The source blocks are the arrows pointing up and the sink blocks are the arrows pointing down. They probably need to have less similar icons but that's where my creativity ended.
The connection for every color needs to be exactly the same length. In the solution above I've already failed this since the orange connection reached the end already at 11 ticks before the rest of the signals are even halfway. Reaching the sink at the same time is the bare minimum to have a valid solution, to get a higher score though the signal integrity needs to be as high as possible.
Signal integrity is highest when the signals are traveling together like in the green/blue line. Every tick every signal is checked for neighbors and if it has a neigboring signal it will increase the quality, if it has two neighboring signals it will increase the quality even more. This is balanced in a way that having no neighbors at all for the signal will quickly drop all the points of the connection so you can only do that for small segments.
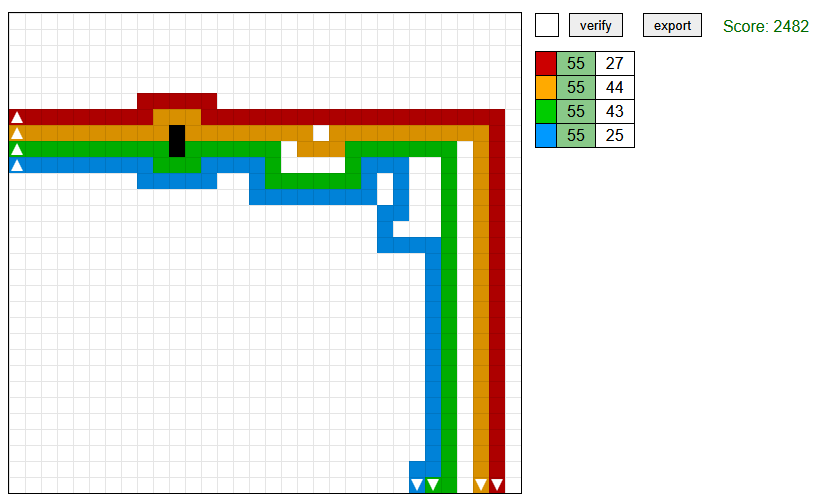
This is a solution to the initial level I made, basically the bare minimum to have a valid solution and it generates 2482 points. This is also the initial version I released on mastodon some days ago so a few people have tried to beat that score, and it has been done easily. The current high score for this level is now 4000 points by @viciouss.
The current dev version of the game can be played at https://brixitcdn.net/game/
Internals
The internals for the game are not terribly exciting. It's mostly full of hooking javascript events to gamecode like this:
const self = this;
this.canvas.addEventListener('mouseenter', function () {
self.hover = true;
self.dirty = true;
});
this.canvas.addEventListener('mouseleave', function () {
self.hover = false;
self.dirty = true;
});
this.canvas.addEventListener('mousemove', function (event) {
const bounds = self.canvas.getBoundingClientRect();
self.hover = true;
self.mouse.x = event.clientX - bounds.left;
self.mouse.y = event.clientY - bounds.top;
self.dirty = true;
if (self.mouseHeld) {
let mouse = [Math.floor(self.mouse.x / 16), Math.floor(self.mouse.y / 16)];
if (mouse[0] !== self.lastMouse[0] || mouse[1] !== self.lastMouse[1]) {
self.lastMouse = mouse;
self.click(mouse[0], mouse[1]);
}
}
});
The game uses a dirty
flag to repaint the game only when there's actual changes, because on the naive requestAnimationFrame()
approach the thing still pins an entire core just drawing a basic grid for some reason.
The entire state of the game is stored in a simple two dimensional array where the contents are either null or an TCBlock()
object that stores the color, type and state of that pixel. Most the code in the game deals with implementing drawing lines inside this array.
When the verify button is pressed a loop is started where every 200ms the entire playfield is simulated. On the initial step all the source blocks will get a "charge" and then every step that charge gets propagated in all cardinal directions if a block of the same color exists in that location. The game tracks every pixel that has had a charge before so it is not possible to create an infinite loop with this mechanic. The simulation terminates when either all charges have been absorbed by a sink block or a simulation step doesn't change the state of the game anymore.
The most interesting part is the loading/saving system. I wanted to have the entire level stored in the URL so it's easy to share levels made in the game. This way I also don't need any server side code and the entire thing can just be static HTML, CSS and javascript.
My initial implementation of saving the game was exporting the state array to JSON and then base64 encoding that to an URL fragment. It turns out that this already consumes 400 characters for just the initial level with 8 blocks on it set. The size explodes when there's actually lines drawn in the level.
My first fix for this was adding brotli.js into the codebase and compressing the json blob before encoding it as base64. This helps a lot and brings the size down to 80 characters for the initial state, but it's still way too large. The solution is obviously to use some actual engineering instead of just throwing things into JSON.stringify here.
There are only four numbers that need to be saved for every block that is set:
- The X and Y coordinate
- The color, every block has a hex string as color.
- The block type: "source", "sink", "wire", "wall".
For the color I added a lookup table to the game and defined a series of colors so I can just store an index into that array. For the block type I simply stored a single character for the types: "S", "s", "w" and "o" for the four block types. The coordinates are also never larger than 256 in any direction so this means I can pack every block into 4 bytes. With this improvement my initial state was down to 32 bytes and with my test solution it was around 200 bytes.
I decided to throw brotli against this problem again to fix this. The initial state of 32 bytes gets compressed with brotli to... 36 bytes... so that doesn't help at all. But the 200 byte field gets compressed to ~100 bytes which is a nice improvement. It can still be better though...
I don't actually need that many colors, I probably don't need more than 4 types in total and the coordinates are nowhere near 256. I slightly shrunk the play area from 640x480 to 512x480 so the largest coorinate in my 16x16 grid becomes 32. This means I can pack the X and Y coorinate together in just 10 bits. If I limit the other values to 8 maximum I can store that in 6 bits together giving me the state for a single block packed into 2 bytes instead of 4, halving the size of the savegames again.
This is what's now actually in use in the demo to save the initial states and what makes the "export" button work that puts your entire solution in the URL.
const buf = new ArrayBuffer(result.length * 2);
const bufView = new Uint8Array(buf);
result.forEach((item, i) => {
bufView[(i * 2)] = (item[0] & 0x1F) | (item[2] << 5);
bufView[(i * 2) + 1] = (item[1] & 0x1F) | (item[3] << 5);
});
console.log("Packed:", buf.byteLength);
const compressed = brotli.compressArray(bufView, 11);
console.log("Compressed:", compressed.length);
window.location.hash = btoa(String.fromCharCode.apply(null, compressed));
The result
array already contains the data compressed into the [[x,y,colorIdx,typeIdx]]
format so that's run through the byte packing code, the compressor and finally the base64 encoder to produce the savegame.
Conclusion
It was suprisingly easy to make all of this, I have of course already used all the seperate components I've used here before so that saves a lot of research. In total I probably spend less than a day to implement all of this, including writing this blog post.
The game itself seems reasonably fun. It can probably be a bit more difficult and the level editing can certainly be a lot better.
The mechanic that is stolen from PCB design in KiCad seems to work pretty well and the scoring mechanism certainly points you into a solution that closely mirrors the advice you get for routing differential signals: Length match the pairs, add timing wiggles close to the source of the features that change the timing to make the differential signalling work the best.
You can play the game at https://brixitcdn.net/game/ and the level editor is available at https://brixitcdn.net/game/#editor
Source code for this is at https://git.sr.ht/~martijnbraam/TimeCode